Programming
Santiago Calvo • 02 MAY 2023
Prisma: The Developer-Friendly ORM for TypeScript Applications
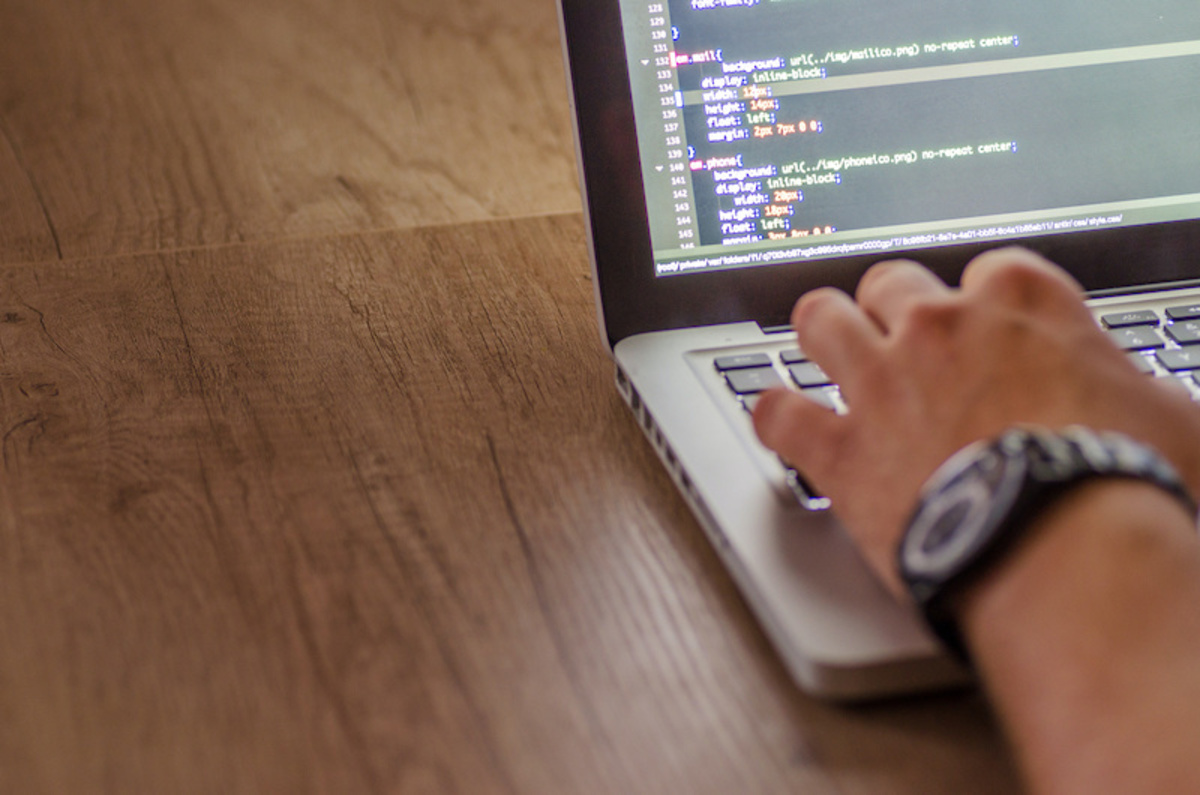
When it comes to startups, speed is of the essence, especially when starting with a minimum viable product (MVP) from scratch. However, when using TypeScript to develop applications, it is equally important to maintain clean types to fully leverage the language's benefits. Unfortunately, maintaining types between external systems can be complex and often involves duplicating efforts.
One such example is dealing with databases. If we use raw SQL connections, we would need to create database migrations that specify types, and then create interfaces or types for each table in the database alongside the types that queries return. All this extra work can slow us down when we need to move quickly.
Ruby on Rails applications, on the other hand, use ActiveRecord to manage database models through migrations, generating new schemas and creating the necessary methods to access each model through Ruby's metaprogramming features. However, in TypeScript, we cannot generate methods on the fly while still being typesafe.
ORMs options: TypeORM, Sequelize and Prisma ORM for nextjs.
To address this, there are several options for Object-Relational Mapping (ORMs) that offer type safety. TypeORM and Sequelize are two popular ones, but today we will be focusing on Prisma ORM for nextjs. This ORM takes a different approach compared to the others mentioned above.
Understanding Prisma: An Introduction to the Innovative ORM and Its Workflow
Prisma is an open-source ORM that prioritizes developer experience (DX) over other ORMs. It provides a seamless and typesafe interface to access databases, allowing developers to focus on building their application's business logic instead of spending time writing boilerplate code.
Unlike other ORMs, Prisma consists of three parts:
- The Prisma client
- Prisma migrate
- Prisma studio.
Its toolset not only includes an ORM but also code generation tools that create migrations and types for our application, allowing us to concentrate on developing features.
So, what exactly do we write in Prisma if it already generates the types and migrations for us? We modify the schema with its intuitive syntax. This schema then generates the necessary migrations to modify the database to reflect the schema. Additionally, it generates the types for our Prisma client.
The three parts of Prisma
1 - The Prisma client is an auto-generated library for Node.js that is responsible for communicating with the database. The client methods are generated based on the schema and are automatically typesafe. This means that developers can write less code and avoid mistakes that could arise from manual type-checking.
2 - Prisma ORM for nextjs migrate, as its name implies, is responsible for handling database migrations. It generates SQL commands to update the schema and keep the database up to date. This means 0that developers don't have to worry about the nitty-gritty details of database migration and can instead focus on writing code.
3 - Prisma studio is a web-based tool that provides a GUI to interact with the database. It allows developers to view the data in the database, modify the schema, and execute queries.
In summary, Prisma is an ORM that streamlines the development process by generating types and migrations based on the schema. Its intuitive syntax and code generation tools reduce boilerplate code and make it easier to work with databases. The Prisma client is typesafe, the migrate tool handles database migrations, and Prisma studio provides a web-based interface to the database. Together, they bring us a seamless and enjoyable development experience.
Using Prisma ORM for nextjs in Action: A Quick Example
Prisma's capabilities sound impressive, but do they live up to the developer experience they promise? Let's explore this by taking a quick example.
1- To start, we need to install the required dependencies and generate the Prisma files:
npm install prisma
npx prisma init
This will create a folder called "prisma" with a schema.prisma file inside, and a .env file in the root folder. We can modify the schema.prisma file to define our User model with various fields, such as id, name, email, and timestamps for createdAt and updatedAt
datasource db {
provider = "postgresql"
url = env("DATABASE_URL")
}
generator client {
provider = "prisma-client-js"
}
model User {
id Int @id @default(autoincrement())
name String
email String @unique
createdAt DateTime @default(now())
updatedAt DateTime @updatedAt
}
2- Now we can generate a Prisma client by running a command:
npx prisma generate
npx prisma migrate dev --name init
This generates a client that we can use to interact with our database. We can also use the migrate command to create a new migration named "init," which generates the necessary tables and types needed to continue.
3- With these steps completed, we can write a TypeScript file that uses our Prisma client to create a new user:
import { PrismaClient } from '@prisma/client'
const prisma = new PrismaClient()
async function main() {
const user = await prisma.user.create({
data: {
name: 'John Doe',
email: 'john.doe@example.com',
},
})
console.log(user)
}
main()
.catch((e) => console.error(e))
.finally(async () => {
await prisma.$disconnect()
})
We can import the PrismaClient from the @prisma/client package and create a new instance of the client. Then, we can use the user.create method to create a new user in our database.
When we run this TypeScript file, it will create a new user in our database and log the user object to the console.
{
id: 1,
name: 'John Doe',
email: 'john.doe@example.com',
createdAt: 2023-03-13T14:03:46.046Z,
updatedAt: 2023-03-13T14:03:46.046Z
}
This example demonstrates how Prisma simplifies the process of writing an MVP by reducing the time spent on database management, allowing developers to focus on more critical aspects of their projects.
Conclusion
In conclusion, Prisma ORM for nextjs is a powerful and developer-friendly ORM that provides a lot of tools and features, including code generation tools for migrations and types, a Prisma client for interacting with the database, and an intuitive schema syntax. It also has built-in pagination and middleware support, as well as the Prisma Studio for visualizing and editing data.
While Prisma does lack some features that other ORMs have, such as polymorphic associations and single table inheritance, the Prisma team is constantly working on improving the library and adding new features. If these features are not critical for your application, or if you can implement them on the application layer, we highly recommend using Prisma.
Overall, Prisma is a great option for maintaining clean types in TypeScript applications while working with external systems like databases, and it can help you move fast while still being typesafe. We encourage you to explore Prisma further and see how it can benefit your project.
If you’d like to learn more about Typescript applications and Node.js, keep reading our blog!